Fundamental Classes
This section describes the fundamental classes that you will need to interact with when building your custom study/strategy. For a complete view of all of the classes/interfaces in the SDK, please consult the API documentation.
Packages
The SDK consists of the following 6 packages:
com.motivewave.platform.sdk.common – Contains common classes and interfaces. These include ‘info’ classes, enumerations, utility functions and ‘context’ classes that expose functionally and data from MotiveWave™
com.motivewave.platform.sdk.common.desc – Contains ‘Descriptor’ classes. These are used to describe settings and values to the MotiveWave™ runtime environment.
com.motivewave.platform.sdk.common.menu – Contains classes for implementing custom context menus.
com.motivewave.platform.sdk.draw – The classes in this package are used to draw figures on the price and study plots.
com.motivewave.platform.sdk.study – Contains the base classes for creating and interacting with studies and strategies.
com.motivewave.platform.sdk.order_mgmt – Contains classes/interfaces for managing orders. These are used in conjunction with strategies.
Study Class
The Study class is the base class for all studies and strategies. When implementing any study/strategy you will first start by deriving directly or indirectly from this class.
Why is there no Strategy Class?
Strategies are a specialized version of a study, in fact most strategies are based (at least in part) on an existing study. If there was a separate Strategy class it would be difficult (if not impossible) to implement a strategy by deriving from an existing study. It is for this reason that the methods and properties that are specific to strategies are included in the Study class.
For most studies there are two methods that you will override:
initialize – The purpose of this method is to describe the user configurable settings for the study and describe the runtime behavior.
calculate – This method calculates the values for the study at the given historical bar.
The following diagram illustrates the basic elements that you need to be concerned with in the Study class. For a complete list of methods and properties, see the API documentation.
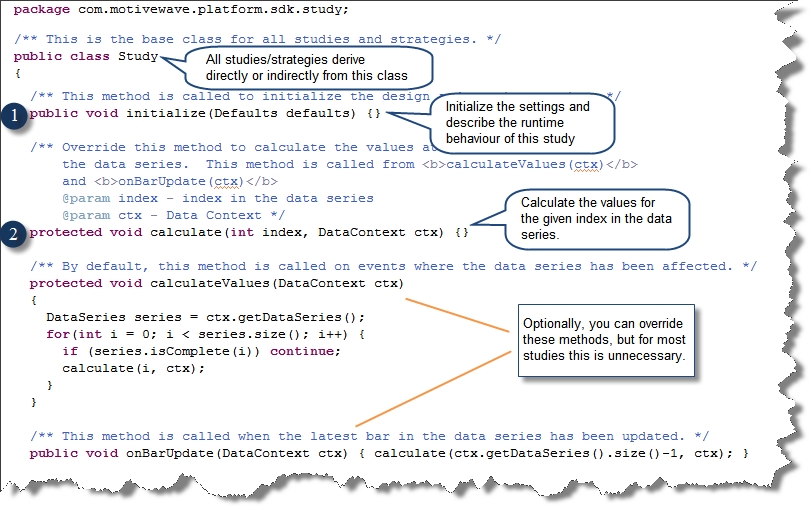
There are 3 main properties in the Study class that are important for implementing a study:
Runtime Descriptor – this describes the runtime behavior of the study
Settings Descriptor – This describes the user settings
getSettings() – This is typically used in the calculate method to get access to the settings that the user has chosen.
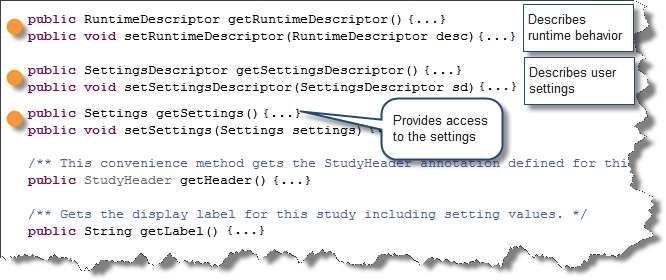
StudyHeader
The StudyHeader is an annotation that is required on every class derived from the Study class. The purpose of this annotation is to describe static information about the study/strategy.
The StudyHeader is read when the Study class is first loaded and is used to register the study with MotiveWave™ and make it available in the Study menu and the ‘Add Study’ dialog.
The following screen shot shows some of the important properties of the StudyHeader. For a full description of all properties see the API documentation.
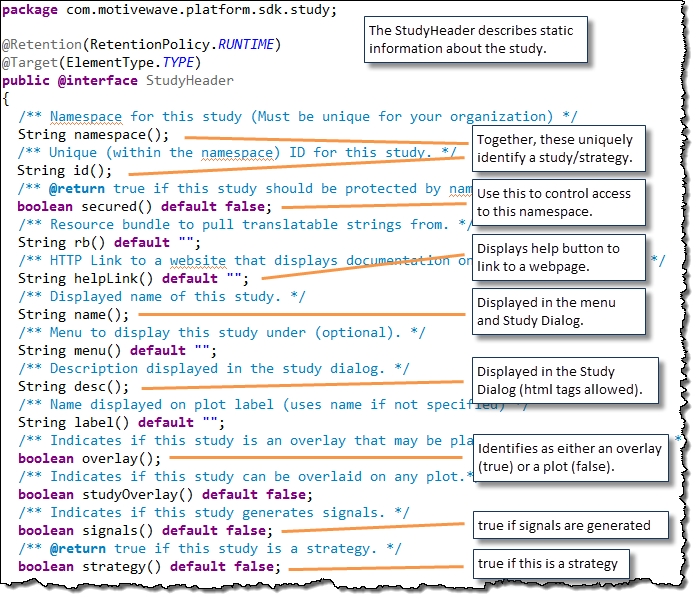
Describing User Settings
The MotiveWave™ SDK provides a lot of flexibility when describing user settings for a study. Settings may be organized into tabs and groups which are displayed in the study dialog. MotiveWave™ also provides many different setting descriptors to represent different types of settings.
The following screen shot illustrates the study dialog for a CCI study:
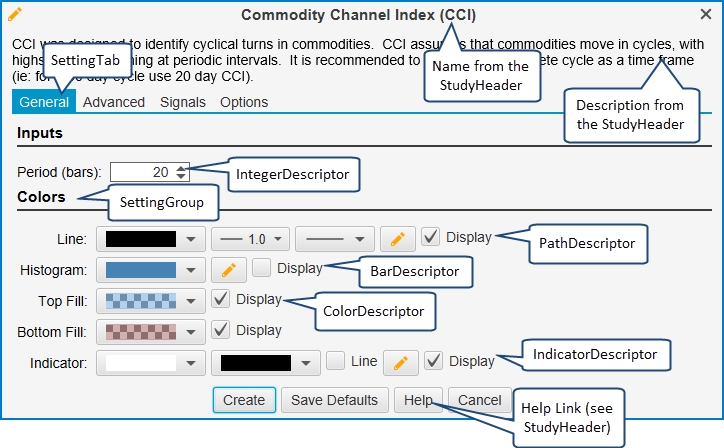
The classes for describing user settings can be found in the package: com.motivewave.platform.sdk.common.desc. The following UML (Universal Markup Language) diagram illustrates the high level classes involved and how they relate to each other. For a full list of the available SettingDescriptor classes, see the API documentation.
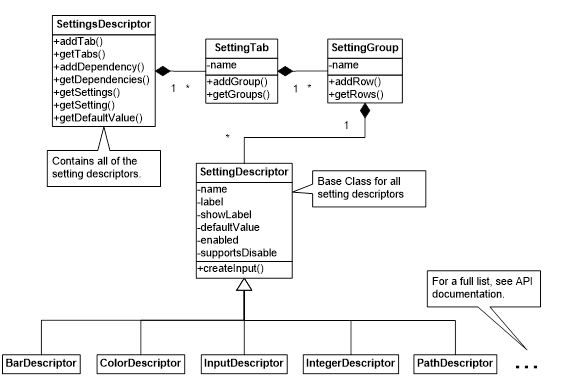
SettingsDescriptor class
The SettingsDescriptor class contains all of the user configurable settings. An instance of this class should be created in the ‘initialize’ method (of the Study class) and assigned to the study using the ‘setSettingsDescriptor’ method.
There are two methods in this class that are important:
addTab – Adds a SettingTab object that contains settings on a tab in the Study Dialog
addDependency – Used to identify dependencies between settings. For example, an ‘EnabledDependency’ will enable a setting if a BooleanSetting is true or false.
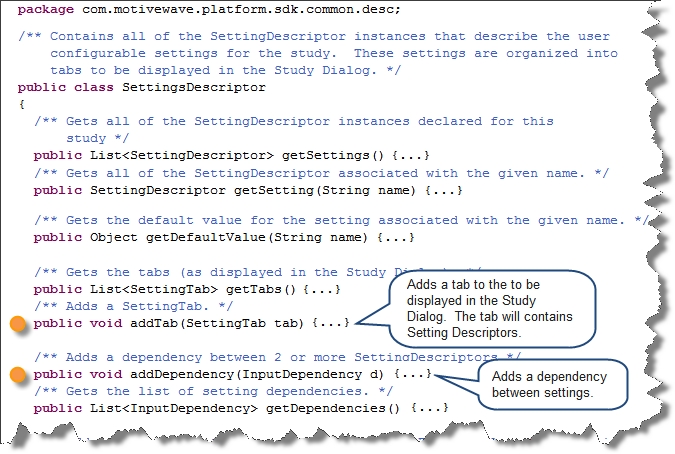
SettingTab Class
The SettingTab class represents a tab in the study dialog. This simple class consists of a name (to display in the tab) and a set of SettingGroup instances.
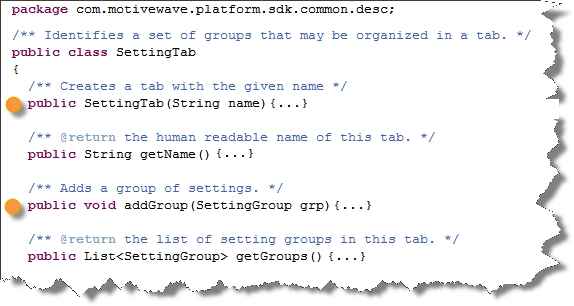
SettingGroup Class
The SettingGroup class organizes related settings into a named group. The group consists of a set of rows that each contains 1 or more setting descriptors.
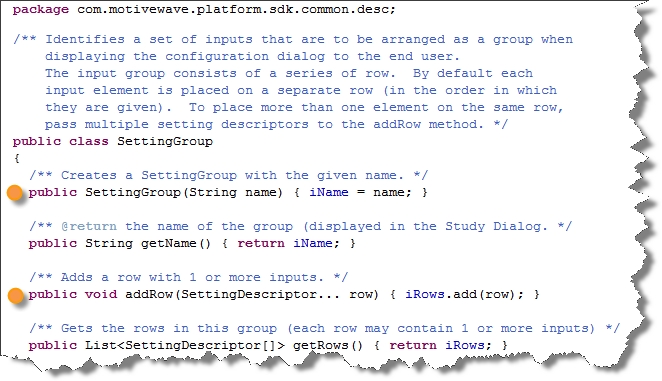
Settings class
The Settings class contains all of the information about the settings configured by the user of the study. You can access this class by using the getSettings() method in the Study base class.
Many of the setting descriptor classes have corresponding ‘Info’ classes (see com.motivewave.platform.sdk.common package) that contain the user specific settings. These may be accessed using a series of ‘get’ methods on the Settings class. The following screen shot illustrates some of these methods. For a complete description of the Settings class and the Info classes see the API documentation.
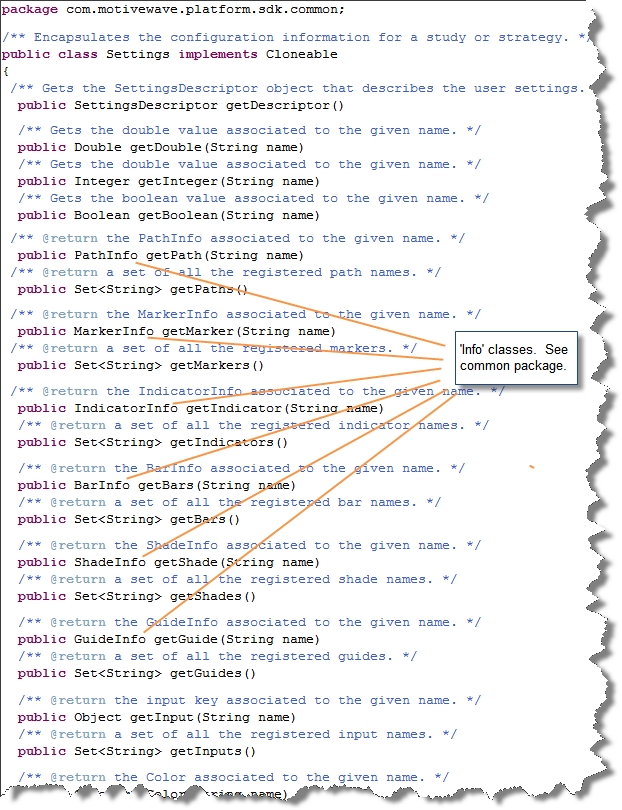
Runtime Settings
The RuntimeDescriptor (com.motivewave.platform.sdk.study package) is used to describe runtime behavior for the study. This includes the following:
Label Settings – used to describe how the label is generated
Export Values – These are values generated by the study that may be used outside of the study.
Declare Elements – These methods associate values generated by the study to visual constructs on the ‘default’ plot (see Composite Studies below for more information):
Paths – A series of values connected by lines
Bars – Vertical bars displayed on a plot
Signals – Signals generated by the study
Indicators – Indicators displayed on the vertical axis
Study Plot Settings (default plot)
Top/Bottom Insets – Used to add space to the top or bottom of the plot
Vertical Range – Range of the vertical axis
Min Tick – precision of the vertical axis values
Horizontal Lines – Horizontal lines displayed on the study plot
Why do I need to declare elements such as a Path?
You may ask yourself, ‘why doesn’t the PathDescriptor (or other descriptor classes) class include the value key?’. While this may make sense in most situations, it does not allow you to use the same path information for multiple paths. Consider for example a case where you have a price bands study and you want to have the same settings for the top and bottom bands. By declaring the path for the top and bottom values as the same path info, you are able to re-use this descriptor object.
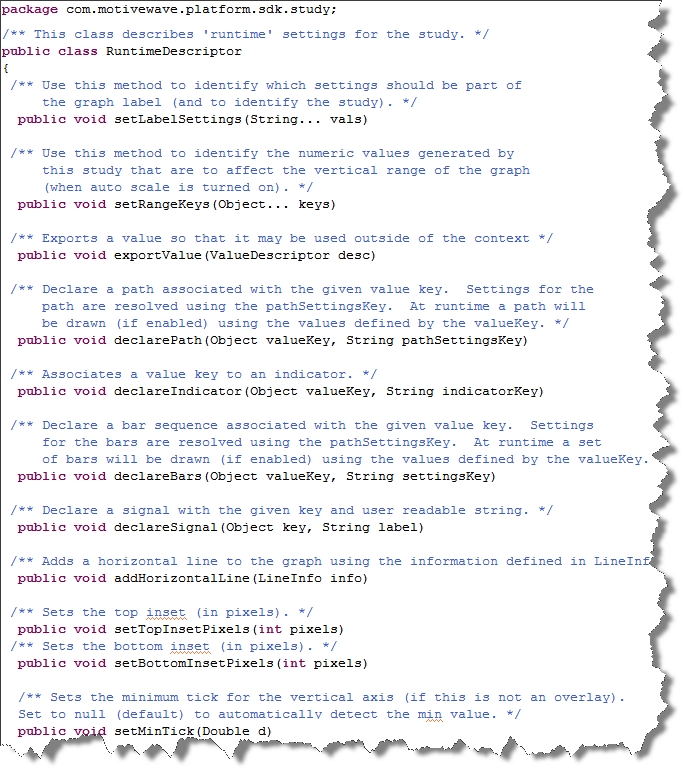
Composite Studies
The majority of studies consist of either a single overlay or a single plot. Version 1.1 of the SDK allows you to create studies that consist of multiple study plots and (optionally) overlays on the price plot.
The RuntimeDescriptor class enables you to define additional plots for a study. This class has been enhanced in version 1.1 to allow the definition of additional plots using the new Plot class (see com.motivewave.platform.sdk.study package).
The majority of methods on the RuntimeDescriptor class operate on the ‘default’ plot for the study. In the case of an overlay, the default plot will be the plot where the overlay was added. For example, when you add a simple moving average (SMA) to the price plot, the default plot for the overlay will be the price plot.
Additional plots may be defined using the Plot class. Each plot has independent settings for labels, tabs, range keys etc and elements are declared separately for each plot (ie paths, bars etc). The following diagram illustrates the relationship between the RuntimeDescriptor and the Plot classes.
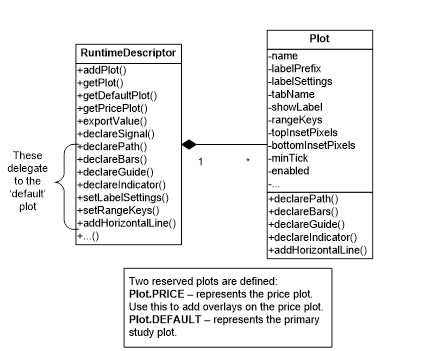
DataContext Interface
The DataContext interface provides access to historical data as well as utility methods for interacting with the study framework.
The following diagram illustrates some of the useful methods:
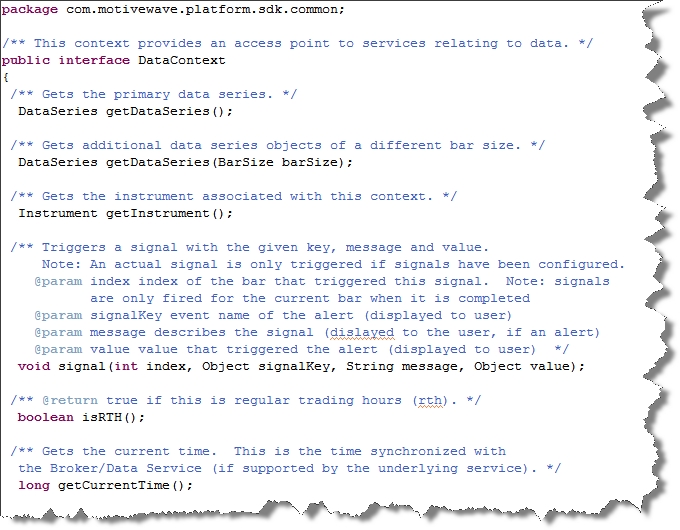
DataSeries Interface
The primary objective of the DataSeries interface is to provide a repository for historical price data and data generated by the study. Data stored in this interface is accessed by a numerical index which represents the price bar where the data applies.
The following diagram illustrates the structure of the data in the data series. Essentially the data is an array of tables where the index ‘0’ is the first (oldest) bar and index ‘size()-1’ is the latest bar.
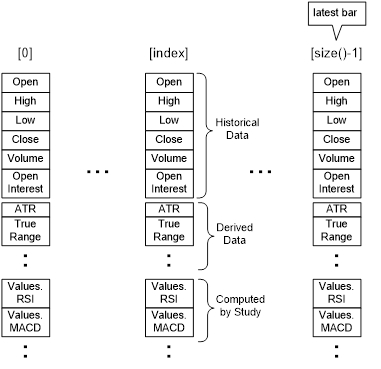
The DataSeries interface also contains a number of convenience methods for calculating common values such as moving averages, swing points and lowest or highest values.
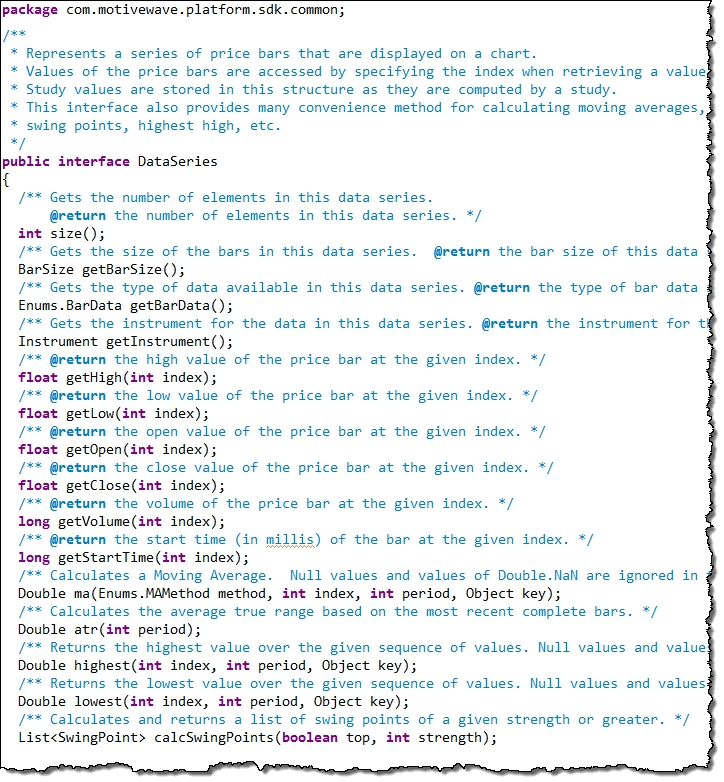
Multiple Instruments
Version 1.1 of the SDK offers support for multiple instruments. This allows you to retrieve real time and historical data for one or more instruments (beyond the primary instrument) for studies and strategies. For strategies you may also place orders for multiple instruments (see section on strategies).
Please Note: Not all editions of MotiveWave™ include support for multiple instruments. In these cases, studies requiring multiple instruments will not be accessible to the end user.
Design Time
Usage of multiple instruments requires the declaration of this feature in the StudyHeader and usage of the InstrumentDescriptor to declare the instruments that will be used at run time.
There are essentially two items that are necessary to enable multiple instruments as part of the design time:
Declare support for multiple instruments – In the StudyHeader set the attribute multipleInstrument=true
Declare one or more instruments in the initialize() method – Use the InstrumentDescriptor to declare one or more instruments. For details on how to use this class, see the API documentation.
The following code snippet illustrates the usage of the ‘multipleInstrument’ attribute in the built-in Spread study:
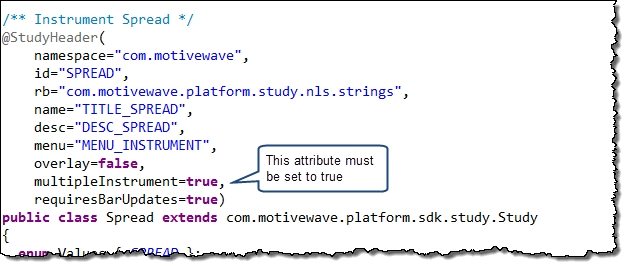
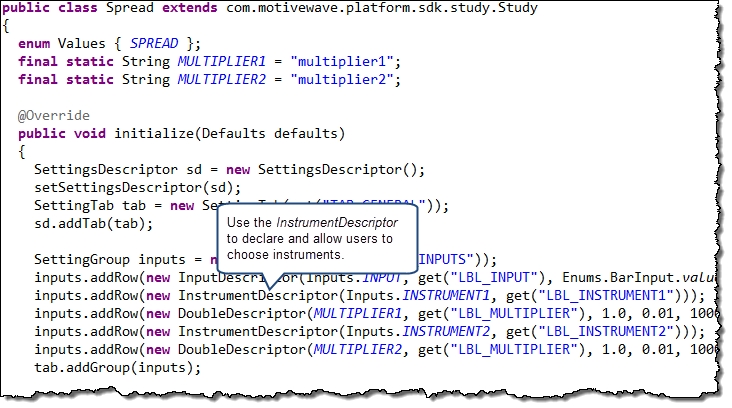
The following screen shot demonstrates how the InstrumentDescriptor enables the user to choose the instrument when they create the study
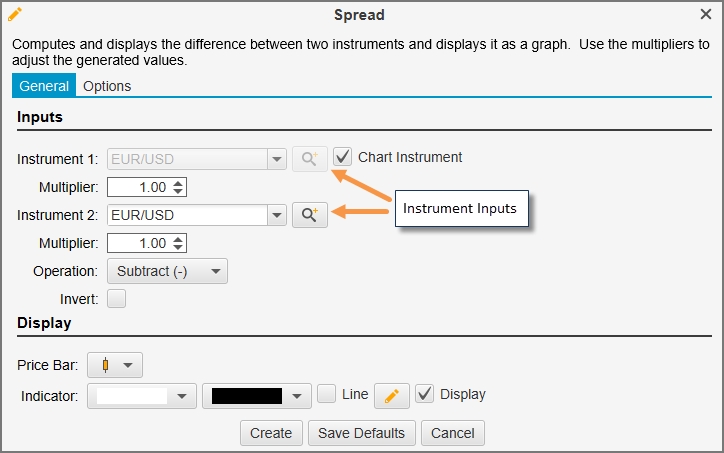
Run Time
Several enhancements have been added to the SDK to enable access settings and historical/real time information in the run time portion of the study:
Settings – a new method getInstrument(key) on the Settings class allows you to retrieve the instrument that the user chose when they created (or modified) the study.
DataSeries – several new methods have been added to the DataSeries interface for retrieving information. Essentially, these are overloaded methods of getDouble(…), getHigh(…), getLow(…) getClose(…) etc.
The following code snippet from the Spread study shows how to retrieve chosen instruments and historical data from the DataSeries interface:
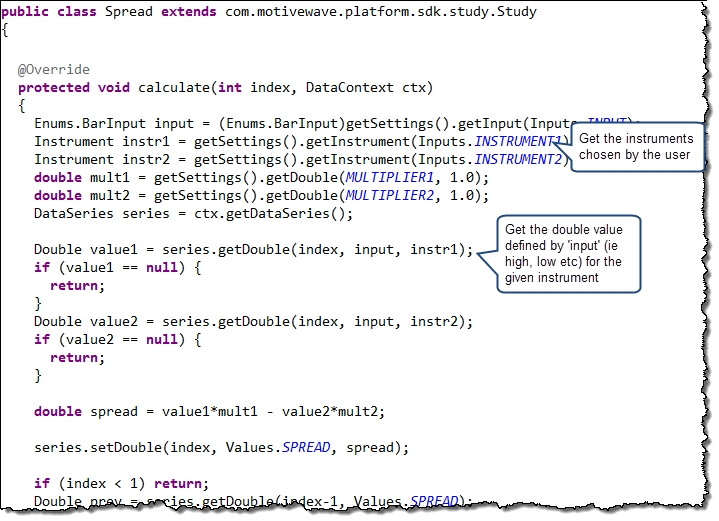
Custom Context Menu
Support for custom context menus was added in version 5.3 of MotiveWave. This feature enables a user to interact with a study without having to open the study dialog. The following screen shot shows an example of a custom context menu in the Trend Line study example. In this example two additional items have been added to the context menu:
Extend Left – Extends the trend line to the left of the screen
Extend Right – Extends the trend line to the right of the screen
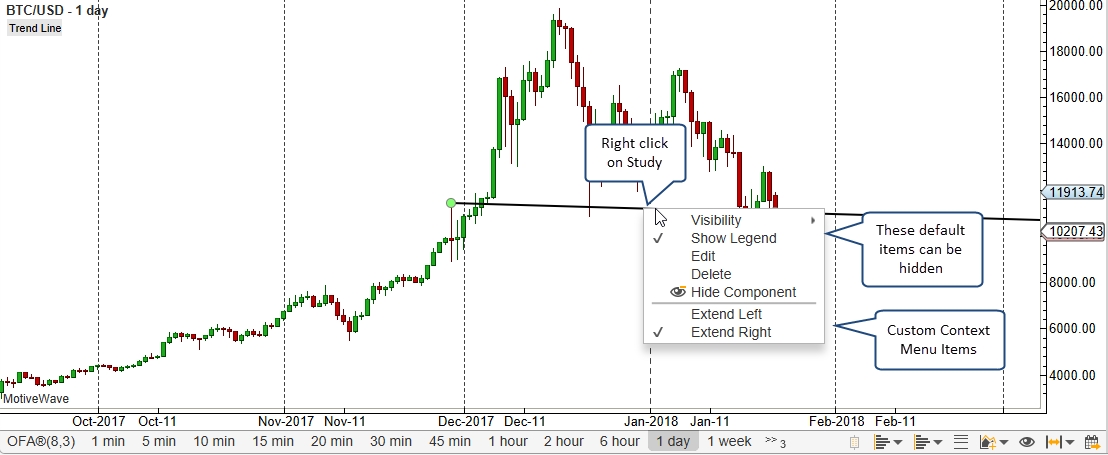
The following excerpt from the TrendLine example study class demonstrates how to add custom menu items. You can use the “plotName” (for composite studies) and “loc” parameters to customize the items depending on where the context menu is requested (where the user does the right click).
Whenever a menu item is invoked the study is recalculated. Typically the ‘action’ part of the menu item is to modify a setting in the study. When the study is recalculated, it will pick up the change to the study settings.
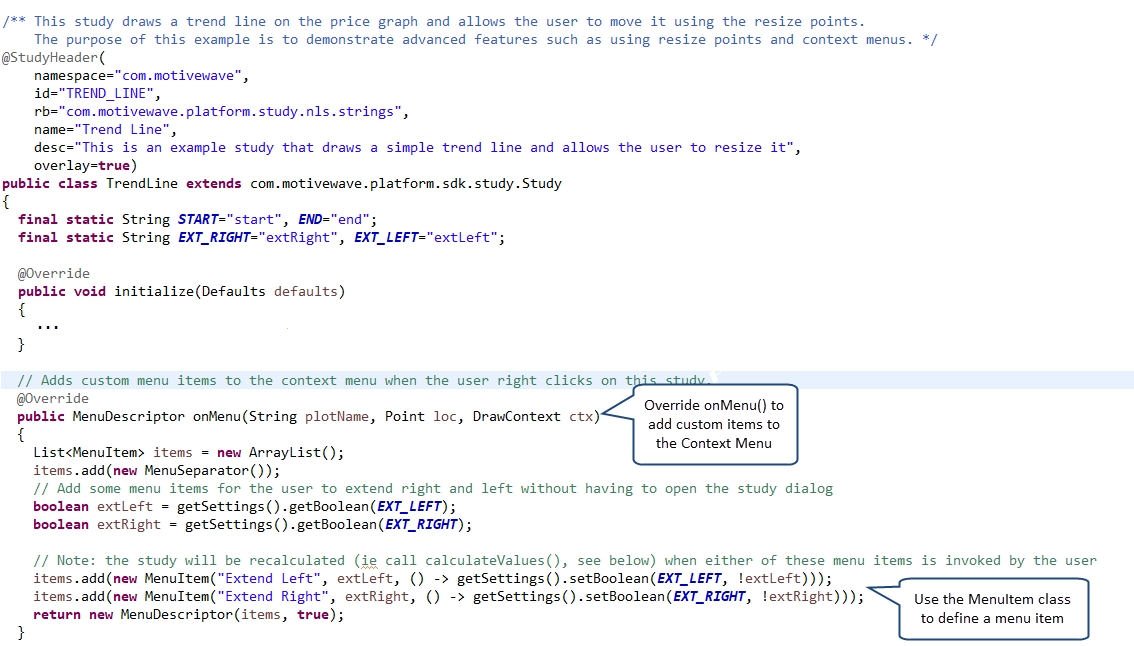
The following diagram illustrates the classes used to define custom context menus for a study. Submenus can be created by using the Menu class (which contains a list of MenuItem, ie ‘items’). The MenuSeparator class may be used to add dividers to the menu. Finally the MenuDescriptor class is used to describe the context menu. Use the ‘includeDefaultItems’ to show or hide the default menu items that are displayed as part of the context menu.
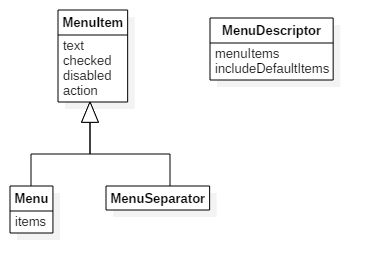
Miscellaneous Classes
The following diagram illustrates some additional classes that may be of interest. These classes are available in the common package (com.motivewave.platform.sdk.common). For full details on these and other classes, please consult the API documentation.
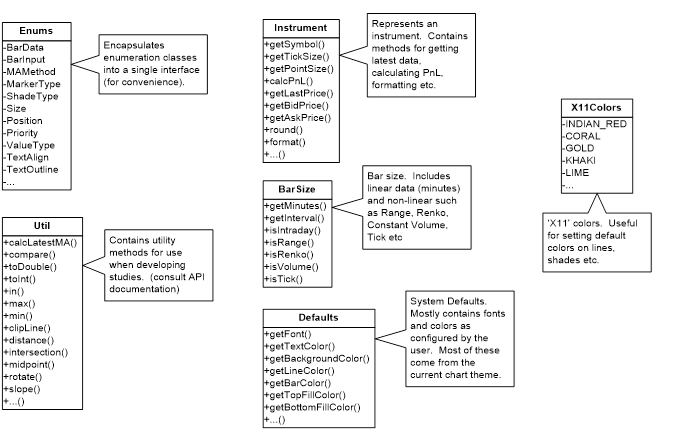
Last updated